REST (Representational State Transfer) is an architectural style used for designing web services that communicate over the internet. It enables applications to exchange data in a simple, scalable, and flexible manner using standard HTTP protocols.
Today, REST is the most widely used architecture for building APIs in modern applications, including web, mobile, and cloud-based services.
In this article, we will explore:
✔ What REST is and why it matters
✔ Key principles of REST architecture
✔ HTTP methods used in RESTful APIs
✔ Real-world examples and use cases
Let’s get started! 🚀
🔹 What is REST?
REST (Representational State Transfer) is a set of architectural principles for designing networked applications. It was introduced by Roy Fielding in 2000 as part of his doctoral dissertation.
In simple terms, REST provides a way for computers to talk to each other over the internet in a stateless and scalable manner using HTTP requests.
💡 Key Features of REST:
✔ Client-Server Architecture — Separates concerns between clients and servers.
✔ Stateless Communication — Each request contains all the information needed.
✔ Cacheability — Responses can be cached to improve performance.
✔ Uniform Interface — Uses a consistent and standardized way to interact with APIs.
✔ Layered System — Supports security, load balancing, and proxies.
REST is widely used in web services because it is simple, scalable, and lightweight.
🔹 How Does REST Work?
RESTful APIs allow applications to communicate using HTTP requests and responses. Clients (such as web browsers or mobile apps) send requests to servers, and the server responds with the requested data.
📌 Example: Requesting User Data from a REST API
📝 Client Request:
GET /users/1 HTTP/1.1
Host: api.example.com
📌 Server Response:
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
The client sends a GET request to fetch user data, and the server responds with a JSON object containing user details.
🔹 Key Principles of REST
To be considered RESTful, an API must follow these six key principles:
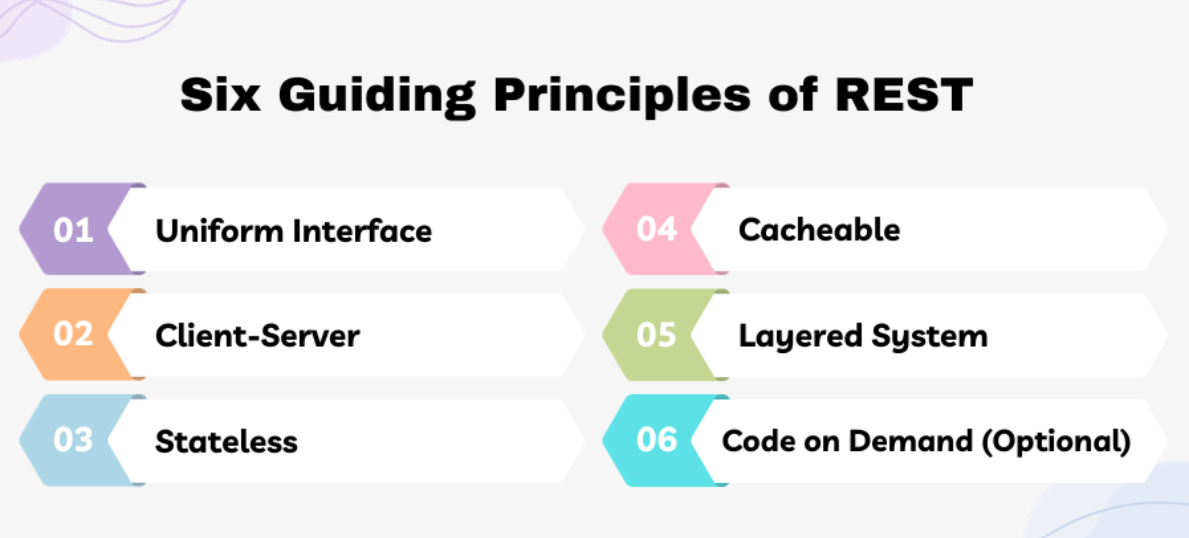
1️⃣ Client-Server Architecture
- The client (e.g., browser, mobile app) sends requests.
- The server (e.g., backend application) processes requests and sends responses.
- This separation improves scalability and flexibility.
2️⃣ Statelessness
- Each request must contain all the necessary information for the server to process it.
- The server does not store client state between requests.
📌 Example: Stateless Request for a Product
GET /products/101 HTTP/1.1
Host: api.example.com
Every request is independent and does not rely on previous requests.
3️⃣ Cacheability
- REST allows caching responses to reduce server load and improve performance.
- The server can specify caching rules using HTTP headers.
📌 Example: Setting a Cache Header
Cache-Control: max-age=3600
This tells the client to cache the response for 1 hour.
4️⃣ Uniform Interface
- REST follows a standardized approach for interacting with resources.
- It uses HTTP methods (
GET
,POST
,PUT
,DELETE
) and resource URIs.
5️⃣ Layered System
- A REST API can have multiple layers (security, load balancing, proxies).
- Clients only interact with the API without worrying about backend logic.
6️⃣ Code on Demand (Optional)
- REST APIs can send executable code (like JavaScript) to clients when needed.
🔹 HTTP Methods in REST
REST APIs use HTTP methods to perform different operations on resources.
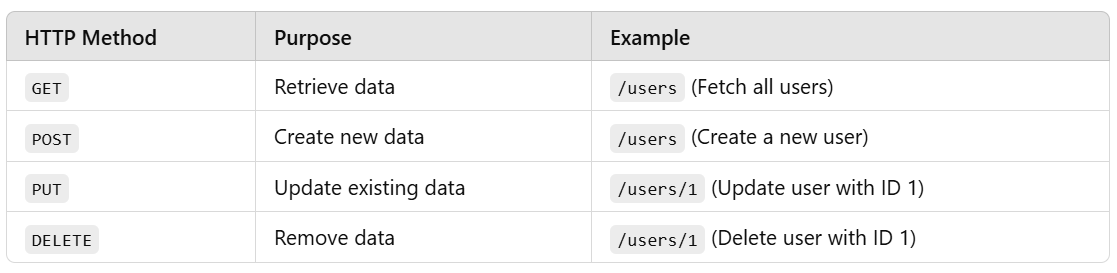
📌 Example: Fetching All Users (GET Request)
GET /users HTTP/1.1
Host: api.example.com
📌 Example: Creating a New User (POST Request)
POST /users HTTP/1.1
Host: api.example.com
Content-Type: application/json
{
"name": "Alice",
"email": "alice@example.com"
}
The POST request sends JSON data to create a new user.
🔹 REST vs. SOAP — What’s the Difference?
SOAP (Simple Object Access Protocol) is another protocol used for web services, but it is heavier and more complex than REST.
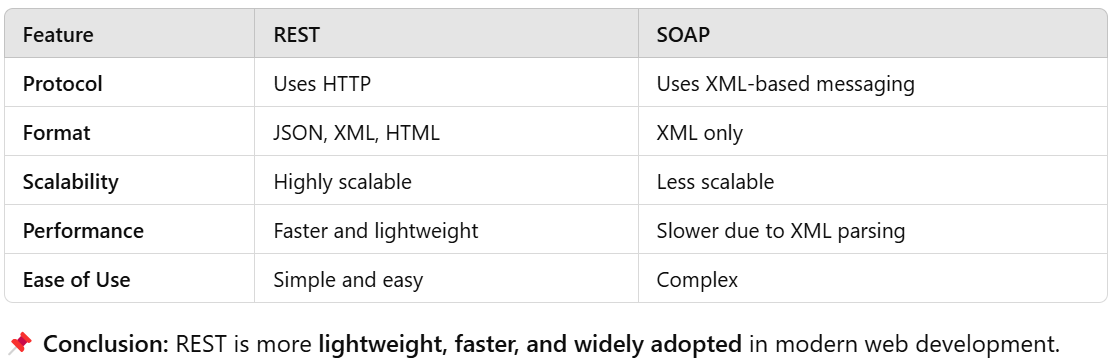
🔹 RESTful API Real-World Use Cases
REST APIs are used in almost every modern application:
✅ Social Media APIs — Facebook, Twitter, and Instagram use REST to fetch user posts and comments.
✅ E-Commerce — Websites like Amazon use REST APIs for product listings, orders, and payments.
✅ Weather Applications — Apps use REST APIs to fetch real-time weather data.
✅ Payment Gateways — PayPal, Stripe, and Razorpay provide REST APIs for transactions.
📌 Example: Using REST API to Fetch Weather Data
GET /weather?q=NewYork&appid=your_api_key
Host: api.openweathermap.org
🚀 The response provides the current weather conditions in New York.
🔹 How to Build a Simple REST API in Python (Flask)
📌 Step 1: Install Flask
pip install flask
📌 Step 2: Create a Simple REST API
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/message', methods=['GET'])
def get_message():
return jsonify({"message": "Hello, REST API!"})
if __name__ == '__main__':
app.run(debug=True)
📌 Step 3: Run the API
python app.py
📌 Step 4: Test the API
GET /api/message HTTP/1.1
🚀 Response: { "message": "Hello, REST API!" }
This demonstrates how easy it is to build a REST API!
🔹 Build REST API using Spring Boot
✅ Spring Boot CRUD REST API Project using IntelliJ IDEA | Postman | MySQL
✅ Spring Boot, MySQL, JPA, Hibernate Restful CRUD API Tutorial
✅ Spring Boot File Upload / Download Rest API Example
✅ Spring Boot, H2, JPA, Hibernate Restful CRUD API Tutorial
✅ Spring Boot Login REST API using Spring Security and MySQL
✅ Login and Registration REST API using Spring Boot, Spring Security, Hibernate, and MySQL Database
✅ Spring Boot REST API Documentation with Swagger
✅ Search REST API using Spring Boot, Spring Data JPA, and MySQL Database
✅ Spring Boot Unit Testing CRUD REST API with JUnit and Mockito
✅ Spring Boot Integration Testing MySQL CRUD REST API Tutorial
🔹 Final Thoughts: Why Use REST?
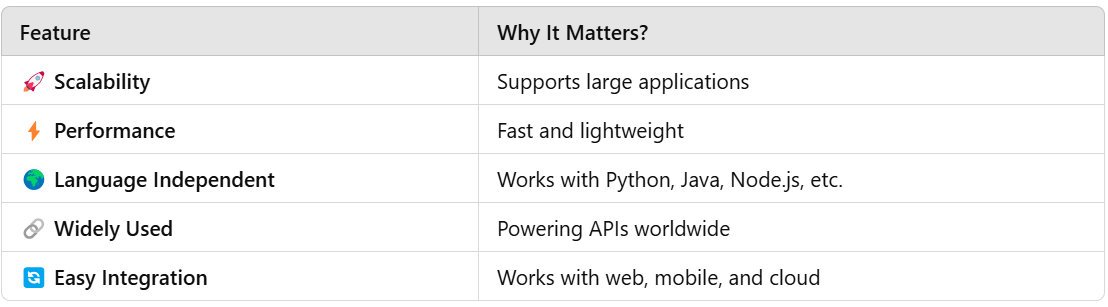
REST is the backbone of modern web services, making it essential for developers.
🚀 Start building RESTful APIs today and power the next-generation applications!
Comments
Post a Comment
Leave Comment